5 Proven Terraform State Management Hacks to Avoid Costly Mistakes
Terraform state is the backbone of Infrastructure as Code (IaC) management with Terraform. It’s essentially a snapshot of your deployed resources, allowing Terraform to track and manage changes efficiently. Understanding and properly managing this state is crucial for avoiding costly errors and ensuring smooth infrastructure operations.
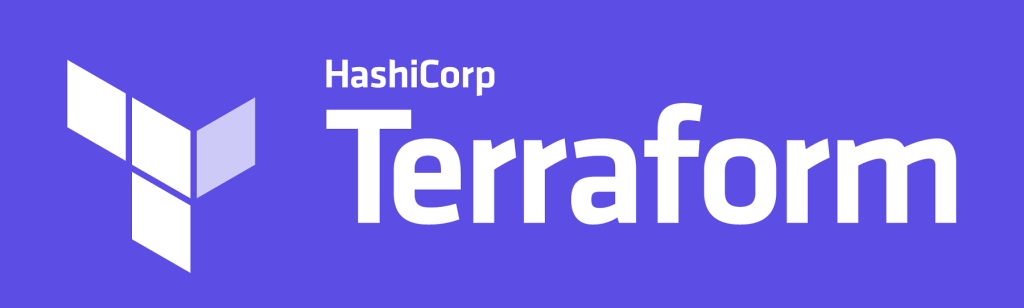
#1: Default Local Backend
Please note that Local Backend is not recommended for most Terraform projects, its useful for testing, but for nearly every circumstance, using remote state is the best option.
When you start using Terraform, it automatically employs a local backend to store the state. This means the state file, typically named terraform.tfstate
, resides on your local machine. While this approach is straightforward for initial exploration, it presents challenges for production environments and collaborative projects.
Limitations of Local State:
- Single Point of Failure: The state file exists only on your machine, creating a risk of loss if your machine fails.
- Collaboration Challenges: Sharing the state file among team members becomes cumbersome and error-prone, potentially leading to inconsistencies and conflicts.
- Scalability Issues: As your infrastructure grows, managing the state file locally can become unwieldy.
#2: Remote State
Terraform supports a variety of remote backends, including cloud storage services and specialized tools. Choosing the right backend depends on your infrastructure and security requirements.
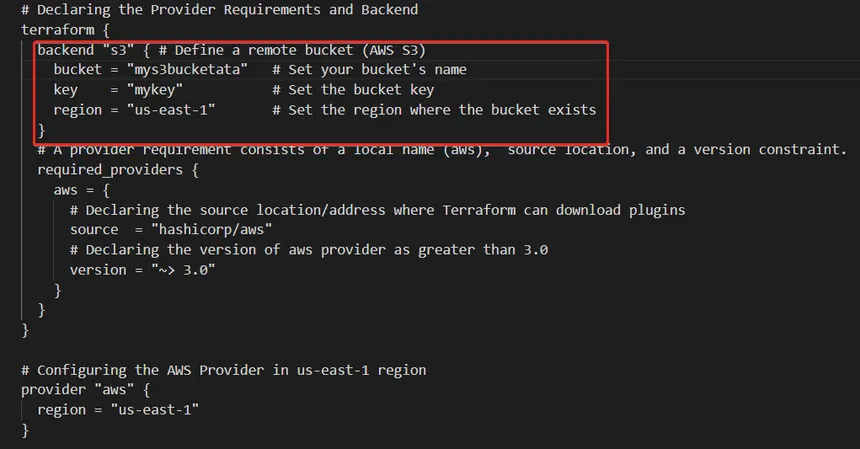
To address the limitations of local state, Terraform offers remote backends. These backends store the state file in a remote location, such as cloud storage services or specialized state management tools.
Popular Backend Options:
- AWS S3: A popular choice for AWS users, offering scalability, durability, and robust security features.
- Azure Storage Account: Ideal for Azure environments, providing secure and reliable storage.
- Google Cloud Storage (GCS): A scalable and cost-effective option for Google Cloud Platform users.
- HashiCorp Consul: A service mesh solution that can also be used for state storage, offering advanced features like locking and versioning.
Benefits of Remote State:
- Centralized Storage: The state file is accessible to all team members from a central location, promoting collaboration and reducing the risk of conflicts.
- Enhanced Security: Remote backends often offer encryption and access control features to protect your state data.
- Improved Reliability: Cloud-based storage provides durability and redundancy, safeguarding your state file from loss.
- Scalability: Remote backends are designed to handle large state files and support growing infrastructure deployments.
Configuring Remote Backends:
Terraform provides a wide array of remote backend options, including AWS S3, Azure Storage Account, Google Cloud Storage, HashiCorp Consul, and more. You configure the backend in your Terraform code using the terraform
block.
Example: AWS S3 Backend
This Terraform code tells Terraform to store its state securely in an Amazon S3 bucket named “my-terraform-state-bucket” located in the “us-west-2” region, using encryption and access control to protect the data.
terraform {
backend "s3" {
bucket = "my-terraform-state-bucket"
key = "my-project/terraform.tfstate"
region = "us-west-2"
# Optional: Encryption and Access Control settings
sse_algorithm = "AES256"
acl = "private"
}
}
Example: Google Cloud Storage Backend
This Terraform code instructs Terraform to store its state in a Google Cloud Storage bucket named “my-terraform-state-bucket” within a folder called “my-project”.
terraform {
backend "gcs" {
bucket = "my-terraform-state-bucket"
prefix = "my-project"
}
}
Example: HashiCorp Consul Backend
Terraform
terraform {
backend "consul" {
address = "consul.example.com"
path = "terraform/state"
}
}
#3: State Locking
State locking is a crucial mechanism for preventing concurrent modifications to the state file, which can lead to corruption and inconsistencies. Terraform automatically implements state locking for operations that could modify the state.
How State Locking Works:
When a Terraform operation that might alter the state is initiated, Terraform attempts to acquire a lock on the state file. If the lock is acquired successfully, the operation proceeds. If another process already holds the lock, Terraform waits until the lock is released.
Disabling and Forcing Unlocks:
You can disable state locking using the -lock
flag for most Terraform commands. However, this is generally not recommended unless you have a specific reason to do so.
In situations where a lock is held by a failed process, you can use the terraform force-unlock
command to release the lock.
#4: Terraform Refresh
The terraform refresh
command serves to synchronize the Terraform state file with the actual state of your deployed infrastructure. It retrieves the latest information about your resources from the infrastructure provider and updates the state file accordingly.
Importance of Refresh:
- Ensuring Accuracy: Over time, the actual state of your infrastructure might drift from the state recorded in the Terraform state file due to manual changes or external factors.
terraform refresh
helps to realign the state file with reality. - Pre-Change Validation: Before applying changes to your infrastructure, it’s crucial to refresh the state to ensure that Terraform has an accurate understanding of the current environment. This helps to avoid unexpected behavior and conflicts during the apply process.
Running Refresh:
You can refresh the state by simply running the following command:
terraform refresh
#5: Sensitive Data in State
Terraform state files can contain sensitive information, such as passwords, API keys, and security tokens. By default, the state file is stored in plain text, posing a security risk.
Protecting Sensitive Data:
- Remote Backends with Encryption: Many remote backends offer encryption capabilities to protect your state data at rest. Configure encryption settings when setting up your remote backend.
- Secret Management Tools: Integrate Terraform with secret management tools like HashiCorp Vault to store and retrieve sensitive data securely.
- Output Variables: Avoid storing sensitive data directly in the state file. Instead, use output variables to retrieve sensitive information from secret management tools or environment variables during runtime.
Example: Using HashiCorp Vault
This Terraform code securely stores a database password in HashiCorp Vault. It then makes that password available to your Terraform project, but hides it from logs and output. Using Vault is important because it keeps your secrets safe and organized, controls who can access them, encrypts them, and can even create temporary secrets that expire to minimize risk. Sources and related content
resource "vault_generic_secret" "db_password" {
path = "secret/data/db-password"
data_json = jsonencode({
"password" = "mysecretpassword"
})
}
output "db_password" {
value = vault_generic_secret.db_password.data["password"]
sensitive = true
}
By implementing these security measures, you can safeguard your sensitive data and maintain the integrity of your Terraform state.
External Links:
- Terraform documentation: https://www.terraform.io/docs/state/index.html
- AWS S3 documentation: https://aws.amazon.com/s3/
- Azure Storage documentation: https://azure.microsoft.com/en-us/products/storage/
- Google Cloud Storage documentation: https://cloud.google.com/storage
- HashiCorp Vault documentation: https://www.vaultproject.io/
Properly managing Terraform state is essential for successful infrastructure automation. By understanding the nuances of local and remote backends, state locking, refresh operations, and security considerations, you can ensure the reliability, consistency, and security of your infrastructure as code.
Recent Comments